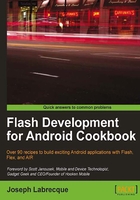
Responding to Android soft-key interactions
AIR for Android does not include support for invoking the native operating system options menu that often appears at the bottom of the screen. However, there are ways of simulating the native behaviour, which we will explore in this section.
The normal behaviour of the back
button, on Android, is to step back through the application states until we arrive back home. A further press of the back
button will exit the application. By default, AIR for Android applications behave in this way as well. If we want to override this default behaviour, we must set up a mechanism to intercept this interaction and then prevent it.
How to do it...
We can respond to soft-key events through standard ActionScript event listeners.
- First, import the following classes into your project:
import flash.display.Sprite; import flash.display.Stage; import flash.display.StageAlign; import flash.display.StageScaleMode; import flash.events.KeyboardEvent; import flash.text.TextField; import flash.text.TextFormat; import flash.ui.Keyboard;
- Declare a
TextField
andTextFormat
object to allow visible output upon the device:private var traceField:TextField; private var traceFormat:TextFormat;
- We will then set up our
TextField
, apply aTextFormat
, and add it to theDisplayList
. Here, we create a method to perform all of these actions for us:protected function setupTextField():void { traceFormat = new TextFormat(); traceFormat.bold = true; traceFormat.font = "_sans"; traceFormat.size = 32; traceFormat.align = "center"; traceFormat.color = 0x333333; traceField = new TextField(); traceField.defaultTextFormat = traceFormat; traceField.selectable = false; traceField.mouseEnabled = false; traceField.width = stage.stageWidth; traceField.height = stage.stageHeight; addChild(traceField); }
- Now we need to set an event listener on the
Stage
to respond to keyboard presses:protected function registerListeners():void { stage.addEventListener(KeyboardEvent.KEY_DOWN, keyDown); }
- We will then write a switch/case statement in our
keyDown
method that will perform different actions in response to specific soft-key events. In this case, we output the name of a specific menu item to ourTextField:
protected function keyDown(e:KeyboardEvent):void { var key:uint = e.keyCode; traceField.text = key + " pressed!\n"; switch(key){ case Keyboard.BACK:{ e.preventDefault(); traceField.appendText("Keyboard.BACK"); break; } case Keyboard.MENU:{ traceField.appendText("Keyboard.MENU"); break; } case Keyboard.SEARCH:{ traceField.appendText("Keyboard.SEARCH"); break; } } }
- The result will appear similar to the following:
How it works...
We register listeners for these Android device soft-keys just as we would for a physical or virtual keyboard in ActionScript. If developing Android applications using AIR for Android, we also have access to the BACK, MENU
, and SEARCH
constants through the Keyboard
class.
Registering a keyboard keyDown
listener and then responding to specific key values through a switch/case statement allows us to respond to the interaction appropriately. For instance, if the MENU
soft-key interaction is detected, we can reveal an options menu.
There's more...
There is also a HOME
soft-key on Android devices. This key press cannot be captured through ActionScript as it exists solely to return the user to the Android home screen from any opened application.
Note
We must use the keyDown
event when we want to cancel the default Android behavior of the BACK
key because the keyUp
event will fire too late and not be caught at all.