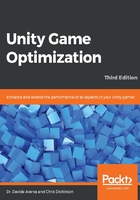
Avoiding re-parenting transforms at runtime
In earlier versions of Unity (version 5.3 and older), the references to Transform components would be laid out in memory in a generally random order. This meant that iteration over multiple Transform components was fairly slow due to the likelihood of cache misses. The upside was that re-parenting GameObject to another one wouldn't really cause a significant performance hit since the Transforms operated a lot like a heap data structure, which tend to be relatively fast at insertion and deletion. This behavior wasn't something we could control, and so we simply lived with it.
However, since Unity 5.4 and beyond, the Transform component's memory layout has changed significantly. Since then, a Transform component's parent-child relationships have operated more like dynamic arrays, whereby Unity attempts to store all Transforms that share the same parent sequentially in memory inside a pre-allocated memory buffer and are sorted by their depth in the Hierarchy window beneath the parent. This data structure allows for much, much faster iteration across the entire group, which is particularly beneficial to multiple subsystems such as physics and animation.
The downside of this change is that if we re-parent GameObject to another one, the parent must fit the new child within its pre-allocated memory buffer as well as sorting all of these Transforms based on the new depth. Also, if the parent has not pre-allocated enough space to fit the new child, then it must expand its buffer to be able to fit the new child, and all of its children, in depth-first order. This could take some time to complete for deep and complex GameObject structures.
When we instantiate a new GameObject through GameObject.Instantiate(), one of its arguments is the Transform component we wish to parent GameObject to, which is null by default and which would place Transform at the root of the Hierarchy window. All Transforms at the root of the Hierarchy window need to allocate a buffer to store its current children as well as those that might be added later (child Transforms do not need to do this). But, if we then re-parent Transform to another one immediately after instantiation, then it discards the buffer we just allocated! To avoid this, we should provide the parent Transform argument into the GameObject.Instantiate() call, which skips this buffer allocation step.
Another way to reduce the costs of this process is to make root Transform pre-allocate a larger buffer before we need it so that we don't need to both expand and re-parent another GameObject instance into the buffer in the same frame. This can be accomplished by modifying a Transform component's hierarchyCapacity property. If we can estimate the number of child Transforms the parent will contain, then we can save a lot of unnecessary memory allocations.