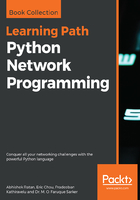
Installation and preparation
The installation instructions for each of the operating systems can be found on the Installing Junos PyEZ (https://www.juniper.net/techpubs/en_US/junos-pyez1.0/topics/task/installation/junos-pyez-server-installing.html) page. We will show the installation instructions for Ubuntu 16.04.
The following are some dependency packages, many of which should already be on the host from running previous examples:
$ sudo apt-get install -y python3-pip python3-dev libxml2-dev libxslt1-dev libssl-dev libffi-dev
PyEZ packages can be installed via pip. Here, I have installed for both Python 3 and Python 2:
$ sudo pip3 install junos-eznc
$ sudo pip install junos-eznc
On the Juniper device, NETCONF needs to be configured as the underlying XML API for PyEZ:
set system services netconf ssh port 830
For user authentication, we can either use password authentication or an SSH key pair. Creating the local user is straightforward:
set system login user netconf uid 2001
set system login user netconf class super-user
set system login user netconf authentication encrypted-password "$1$0EkA.XVf$cm80A0GC2dgSWJIYWv7Pt1"
For the ssh key authentication, first, generate the key pair on your host:
$ ssh-keygen -t rsa
By default, the public key will be called id_rsa.pub under ~/.ssh/, while the private key will be named id_rsa under the same directory. Treat the private key like a password that you never share. The public key can be freely distributed. In our use case, we will move the public key to the /tmp directory and enable the Python 3 HTTP server module to create a reachable URL:
$ mv ~/.ssh/id_rsa.pub /tmp
$ cd /tmp
$ python3 -m http.server
Serving HTTP on 0.0.0.0 port 8000 ...
From the Juniper device, we can create the user and associate the public key by downloading the public key from the Python 3 web server:
netconf@foo# set system login user echou class super-user authentication load-key-file http://192.168.24.164:8000/id_rsa.pub
/var/home/netconf/...transferring.file........100% of 394 B 2482 kBps
Now, if we try to ssh with the private key from the management station, the user will be automatically authenticated:
$ ssh -i ~/.ssh/id_rsa 192.168.24.252
--- JUNOS 12.1R1.9 built 2012-03-24 12:52:33 UTC
echou@foo>
Let's make sure that both of the authentication methods work with PyEZ. Let's try the username and password combination:
Python 3.5.2 (default, Nov 17 2016, 17:05:23)
[GCC 5.4.0 20160609] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from jnpr.junos import Device
>>> dev = Device(host='192.168.24.252', user='netconf', password='juniper!')
>>> dev.open()
Device(192.168.24.252)
>>> dev.facts
{'serialnumber': '', 'personality': 'UNKNOWN', 'model': 'olive', 'ifd_style': 'CLASSIC', '2RE': False, 'HOME': '/var/home/juniper', 'version_info': junos.version_info(major=(12, 1), type=R, minor=1, build=9), 'switch_style': 'NONE', 'fqdn': 'foo.bar', 'hostname': 'foo', 'version': '12.1R1.9', 'domain': 'bar', 'vc_capable': False}
>>> dev.close()
We can also try to use the SSH key authentication:
>>> from jnpr.junos import Device
>>> dev1 = Device(host='192.168.24.252', user='echou', ssh_private_key_file='/home/echou/.ssh/id_rsa')
>>> dev1.open()
Device(192.168.24.252)
>>> dev1.facts
{'HOME': '/var/home/echou', 'model': 'olive', 'hostname': 'foo', 'switch_style': 'NONE', 'personality': 'UNKNOWN', '2RE': False, 'domain': 'bar', 'vc_capable': False, 'version': '12.1R1.9', 'serialnumber': '', 'fqdn': 'foo.bar', 'ifd_style': 'CLASSIC', 'version_info': junos.version_info(major=(12, 1), type=R, minor=1, build=9)}
>>> dev1.close()
Great! We are now ready to look at some examples for PyEZ.