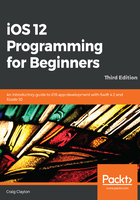
Creating a dictionary
The traditional way of creating a dictionary is to first declare it as a dictionary and then, inside angle brackets, declare a type for the key and value. Let's create our first dictionary inside Playgrounds:

The immutable dictionary we created earlier has a string data type for both its key and value. We have multiple ways to create a dictionary. Let's look at another by adding the following to Playgrounds:
let dictSecondExample = [String: Int]()
Your code should now look like this:

In this latest example, we created another immutable dictionary, with its key having a string data type and its value having an int data type.
If we wanted to use our pizza diagram, the key would have a string data type and the value would have a double data type. Let's create this dictionary in Playgrounds, but, this time, we will make it a mutable dictionary and give it an initial value:
var dictThirdExample = Dictionary<String, Double>(dictionaryLiteral: ("veggie", 14.99), ("meat", 16.99))
Your code should now look like this:

The preceding example is just one way of creating a dictionary for our pizza diagram example. Let's look at a much more common method using type inference:
var dictPizzas = ["veggie": 14.99]
Once you add this to your code, your code should look something like this:

The preceding is a much simpler way of creating a dictionary with an initial value. When initializing a dictionary, it can have any number of items. In our case, we are starting off with just one.
Now, let's look at how we can add more pizzas to our dictionary.