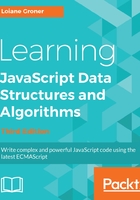
Conditional statements
The first conditional statement we will take a look at is the if...else construct. There are a few ways we can use the if...else construct.
We can use the if statement if we want to execute a block of code only if the condition (expression) is true, as follows:
var num = 1; if (num === 1) { console.log('num is equal to 1'); }
We can use the if...else statement if we want to execute a block of code and the condition is true or another block of code just in case the condition is false (else), as follows:
var num = 0; if (num === 1) { console.log('num is equal to 1'); } else { console.log('num is not equal to 1, the value of num is ' + num); }
The if...else statement can also be represented by a ternary operator. For example, take a look at the following if...else statement:
if (num === 1) { num--; } else { num++; }
It can also be represented as follows:
(num === 1) ? num-- : num++;
Also, if we have several expressions, we can use if...else several times to execute different blocks of code based on different conditions, as follows:
var month = 5; if (month === 1) { console.log('January'); } else if (month === 2) { console.log('February'); } else if (month === 3) { console.log('March'); } else { console.log('Month is not January, February or March'); }
Finally, we have the switch statement. If the condition we are evaluating is the same as the previous one (however, it is being compared to different values), we can use the switch statement:
var month = 5; switch (month) { case 1: console.log('January'); break; case 2: console.log('February'); break; case 3: console.log('March'); break; default: console.log('Month is not January, February or March'); }
One thing that is very important in a switch statement is the use of the case and break keywords. The case clause determines whether the value of switch is equal to the value of the case clause. The break statement stops the switch statement from executing the rest of the statement (otherwise, it will execute all the scripts from all case clauses below the matched case until a break statement is found in one of the case clauses). Finally, we have the default statement, which is executed by default if none of the case statements are true (or if the executed case statement does not have the break statement).