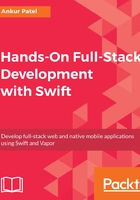
Controlling the flow of our application using View Controller
iOS apps have a controller file, which as the name implies, controls the flow of your application. It's one file that's responsible for keeping track of data that will be used to render the view. It also listens to triggers from the user and reacts to them by modifying the data if needed, and re-rendering the view with the modified data. There are a few kinds of View Controllers, but the one that is most commonly used is a Table View Controller.
Table View Controllers are specialized View Controllers that are used when you want to show a list of data. It can also be used in creative ways to make more complex user interfaces, such as an image reel or a carousel. For our app, we'll use the Table View Controller to list our Shopping List Items one by one, and the View Controller will be responsible for keeping the entire list in memory.
Before we dive into the code for our Table View Controller, we need to understand a few concepts about the life cycle events of a View Controller. Let's look at the life cycle:
- All View Controllers start out by having the viewDidLoad method invoked. This method is only called if the View Controller has not loaded before. This is a good place to load data for our application by generating fake Shopping List Items or by reading a Shopping List from disk. We can also make a network request to get the Shopping List Items.
- After this, the viewWillAppear method is called on our View Controller. This gets called every time the view is going to appear but is not 100% visible to the user yet. This is another good place to refresh our data so that the user sees the most up-to-date Shopping List as the user switches between View Controllers.
- Then, viewDidAppear will get called when the view is visible to the user. This is a good place to end any animation or stop a spinner in case data is being loaded over the network and taking some time to load.
- The same methods exist when the view is removed, first by getting a call to the viewWillDisappear method. And finally, by getting a call to viewDidDisappear. These are good methods to save data or they can be ignored, if you prefer that the data for the Shopping List is saved on every change.
Table View Controller, which is a subclass of View Controllers, inherit the following life cycle methods. We can override these methods as needed to fetch and save data. Table View Controllers also have a few more methods that we will examine by first creating a new Table View Controller file in our project. Like we did for the model, we need to do the following:
- Create a new group called Controllers under the ShoppingList folder in your project
- Then create a New File... under the Controllers folder, select a Cocoa Touch Class from the iOS template, and click Next:
- Under Subclass of type select UITableViewController, name the file ItemTableViewController, and make sure Language is set to Swift. Click Next and then click Create in the next dialog:
Xcode should generate the file and it will have some code commented out. We will fill out this class shortly. Now, we will switch files and jump to the Main.storyboard.