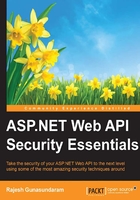
Setting up your browser client
Let's create a Web API for Contact Lookup. This Contact Lookup Web API service will return the list of contacts to the calling client application. Then we will be consuming the Contact Lookup service using the jQuery AJAX call to list and search contacts.
This application will help us in demonstrating the Web API security throughout this book.
Implementing Web API lookup service
In this section, we are going to create a Contact Lookup web API service that returns a list of contacts in the JavaScript Object Notation (JSON) format. The client that consumes this Contact Lookup is a simple web page that displays the list of contacts using jQuery. Follow these steps to start the project:
- Create New Project from the Start page in Visual Studio.
- Select Visual C# Installed Template named Web.
- Select ASP.NET Web Application in the center pane.
- Name the project
ContactLookup
and click OK, as shown in the following screenshot:Fig 2 – We have named the ASP.NET Web Application "ContactLookup"
- Select the Empty template in the New ASP.NET Project dialog box.
- Check Web API and click OK under Add folders and core references, as shown in the following:
Fig 3 – We select the Empty Web API template
We just created an empty Web API project. Now let's add the required model.
Adding a model
Let's start by creating a simple model that represents a contact with the help of the following steps:
- First, define a simple contact model by adding a class file to the Models folder.
Fig 4 – Right-click on the Models folder and Add a Model Class
- Name the class file
Contact
and declare properties of theContact
class.namespace ContactLookup.Models { public class Contact { public int Id { get; set; } public string Name { get; set; } public string Email { get; set; } public string Mobile { get; set; } } }
We just added a model named Contact
. Let's now add the required web API controller.
Adding a controller
HTTP requests are handled by controller objects in Web API. Let's define a controller with two action methods. One action to return the list of contacts and other action to return a single contact specific to a given ID:
- Add the Controller under the Controllers folder in Solution Explorer.
Fig 5 – Right-click on the Controllers folder and Add a Controller
- Select Web API Controller – Empty and click on Add in the Add Scaffold dialog.
Fig 6 – Select an Empty Web API Controller
- Let's name the controller
ContactsController
in the Add Controller dialog box and click Add.Fig 7 – Naming the controller
This creates the
ContactsController.cs
file in the Controllers folder as shown in the following image:Fig 8 – ContactsController is added to the Controllers folder in the application
- Replace the code in
ContactsController
with the following code:namespace ContactLookup.Controllers { public class ContactsController : ApiController { Contact[] contacts = new Contact[] { new Contact { Id = 1, Name = "Steve", Email = "steve@gmail.com", Mobile = "+1(234)35434" }, new Contact { Id = 2, Name = "Matt", Email = "matt@gmail.com", Mobile = "+1(234)5654" }, new Contact { Id = 3, Name = "Mark", Email = "mark@gmail.com", Mobile = "+1(234)56789" } }; public IEnumerable<Contact> GetAllContacts() { return contacts; } public IHttpActionResult GetContact(int id) { var contact = contacts.FirstOrDefault(x => x.Id == id); if (contact == null) { return NotFound(); } return Ok(contact); } } }
For simplicity, contacts are stored in a fixed array inside the controller class. The controller is defined with two action methods. List of contacts will be returned by the GetAllContacts method in the JSON format and the GetContact method returns a single contact by its ID. A unique URI is applied to each method on the controller as given in the following table:

Consuming the Web API using JavaScript and jQuery
In this section, in order to demonstrate calling the web API with or without any security mechanisms, let's create an HTML page that consumes web API and update the page with the results using the jQuery AJAX call:
- In the Solution Explorer pane, right-click on the project and add New Item.
Fig 9 – Select add new item from the context menu in Solution Explorer
- Create HTML Page named
index.html
using the Add New Item dialog.Fig 10 – Add an index html file by selecting HTML page in the Add New Item dialog
- Replace the content of the
index.html
file with the following code:<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Contact Lookup</title> </head> <body> <div> <h2>All Contacts</h2> <ul id="contacts" /> </div> <div> <h2>Search by ID</h2> <input type="text" id="contactId" size="5" /> <input type="button" value="Search" onclick="search();" /> <p id="contact" /> </div> <script src="http://ajax.aspnetcdn.com/ajax/jQuery/jquery-2.0.3.min.js"></script> <script> var uri = 'api/contacts'; $(document).ready(function () { // Send an AJAX request $.getJSON(uri) .done(function (data) { // On success, 'data' contains a list of contacts. $.each(data, function (key, contact) { // Add a list item for the contact. $('<li>', { text: formatItem(contact) }).appendTo($('#contacts')); }); }); }); function formatItem(contact) { return contact.Name + ', email: ' + contact.Email + ', mobile: ' + contact.Mobile; } function search() { var id = $('#contactId').val(); $.getJSON(uri + '/' + id) .done(function (data) { $('#contact').text(formatItem(data)); }) .fail(function (jqXHR, textStatus, err) { $('#contact').text('Error: ' + err); }); } </script> </body> </html>
Getting a list of contacts
We need to send an HTTP GET request to /api/contacts
to get the list of contacts. The AJAX request is sent by the jQuery getJSON
function and the array of JSON objects is received in the response. A callback function in the done
function is called if the request succeeds. In the callback, we update the DOM with the contact information, as follows:
$(document).ready(function () { // Send an AJAX request $.getJSON(uri) .done(function (data) { // On success, 'data' variable contains a list of contacts. $.each(data, function (key, contact) { // Add a list item for the contact. $('<li>', { text: formatItem(contact) }).appendTo($('#contacts')); }); }); });
Getting a contact by ID
To get a contact by ID, send an HTTP GET request to /api/contacts/id
, where id
is the contact ID.
function search() { var id = $('#contactId').val(); $.getJSON(uri + '/' + id) .done(function (data) { $('#contact').text(formatItem(data)); }) .fail(function (jqXHR, textStatus, err) { $('#contact').text('Error: ' + err); }); }
The request URL in getJSON
has the contact ID. The response is a JSON representation of a single contact for this request.
Running the application
Start debugging the application by pressing F5. To search for a contact by ID, enter the ID and click on Search:

Fig 11 – User Interface of the Sample Browser-based Client Application